diff --git a/.idea/vcs.xml b/.idea/vcs.xml
new file mode 100644
index 00000000..94a25f7f
--- /dev/null
+++ b/.idea/vcs.xml
@@ -0,0 +1,6 @@
+
+
+
+
+
+
\ No newline at end of file
diff --git a/.idea/workspace.xml b/.idea/workspace.xml
index d3843bf0..1a77052b 100644
--- a/.idea/workspace.xml
+++ b/.idea/workspace.xml
@@ -1,7 +1,12 @@
-
+
+
+
+
+
+
@@ -17,12 +22,14 @@
-
+
-
-
-
+
+
+
+
+
@@ -40,11 +47,11 @@
-
+
-
+
@@ -54,19 +61,29 @@
-
-
+
+
-
-
-
-
-
-
-
-
-
-
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
@@ -122,6 +139,9 @@
flutter_inappbrowser
+
+
+
-
-
+
+
@@ -161,6 +181,7 @@
+
@@ -380,6 +401,7 @@
+
@@ -398,8 +420,8 @@
-
-
+
+
@@ -456,7 +478,9 @@
-
+
+
+
@@ -724,14 +748,46 @@
-
+
-
-
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
@@ -743,33 +799,13 @@
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
+
-
-
-
+
+
+
+
+
diff --git a/README.md b/README.md
index da4b56ef..1d1bb393 100644
--- a/README.md
+++ b/README.md
@@ -21,37 +21,72 @@ First, add `flutter_inappbrowser` as a [dependency in your pubspec.yaml file](ht
Create a Class that extends the `InAppBrowser` Class in order to override the callbacks to manage the browser events.
Example:
```dart
+import 'package:flutter/material.dart';
+
import 'package:flutter_inappbrowser/flutter_inappbrowser.dart';
class MyInAppBrowser extends InAppBrowser {
-
+
@override
void onLoadStart(String url) {
super.onLoadStart(url);
print("\n\nStarted $url\n\n");
}
-
+
@override
void onLoadStop(String url) {
super.onLoadStop(url);
print("\n\nStopped $url\n\n");
}
-
+
@override
void onLoadError(String url, String code, String message) {
super.onLoadStop(url);
print("\n\nCan't load $url.. Error: $message\n\n");
}
-
+
@override
void onExit() {
super.onExit();
print("\n\nBrowser closed!\n\n");
}
-
+
}
MyInAppBrowser inAppBrowser = new MyInAppBrowser();
+
+void main() => runApp(new MyApp());
+
+class MyApp extends StatefulWidget {
+ @override
+ _MyAppState createState() => new _MyAppState();
+}
+
+class _MyAppState extends State {
+
+ @override
+ void initState() {
+ super.initState();
+ }
+
+ @override
+ Widget build(BuildContext context) {
+ return new MaterialApp(
+ home: new Scaffold(
+ appBar: new AppBar(
+ title: const Text('Flutter InAppBrowser Plugin example app'),
+ ),
+ body: new Center(
+ child: new RaisedButton(onPressed: () {
+ inAppBrowser.open("https://flutter.io/");
+ },
+ child: Text("Open InAppBrowser")
+ ),
+ ),
+ ),
+ );
+ }
+}
```
### InAppBrowser.open
@@ -199,7 +234,7 @@ Injects JavaScript code into the `InAppBrowser` window. (Only available when the
Example:
```dart
inAppBrowser.injectScriptCode("""
-alert("JavaScript injected");
+ alert("JavaScript injected");
""");
```
@@ -222,9 +257,9 @@ Injects CSS into the `InAppBrowser` window. (Only available when the target is s
Example:
```dart
inAppBrowser.injectStyleCode("""
-body {
- background-color: #3c3c3c;
-}
+ body {
+ background-color: #3c3c3c;
+ }
""");
```
@@ -237,3 +272,10 @@ Example:
inAppBrowser.injectStyleFile("https://stackpath.bootstrapcdn.com/bootstrap/4.1.3/css/bootstrap.min.css");
```
+## Screenshots:
+
+iOS:
+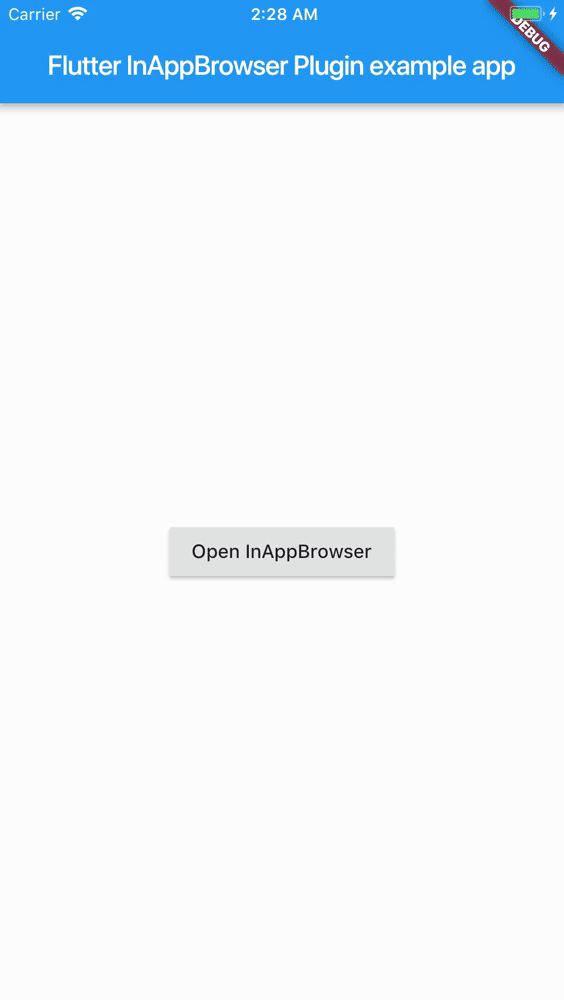
+
+Android:
+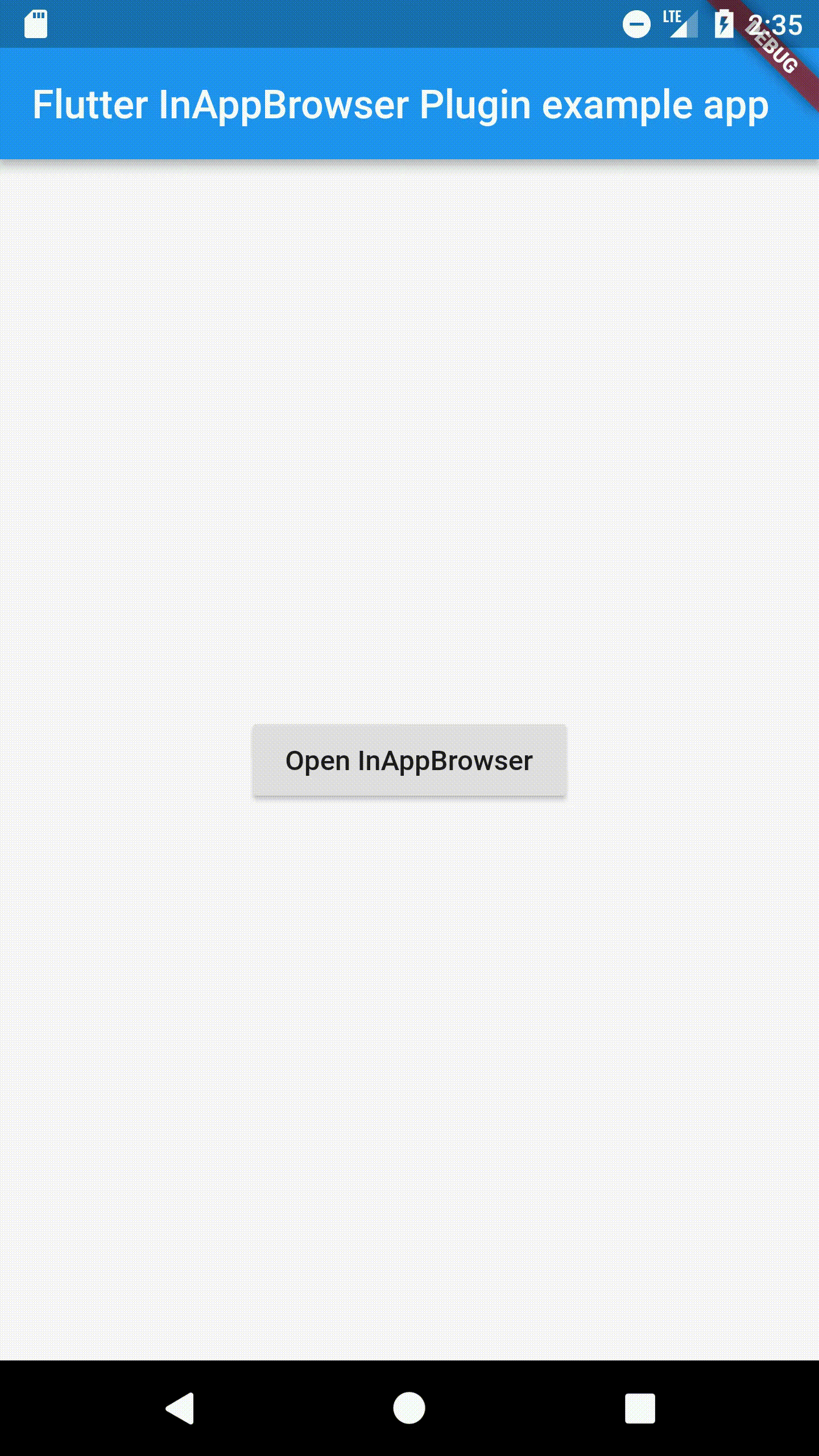
diff --git a/example/lib/main.dart b/example/lib/main.dart
index 50952435..68d82e4c 100644
--- a/example/lib/main.dart
+++ b/example/lib/main.dart
@@ -61,7 +61,7 @@ class _MyAppState extends State {
return new MaterialApp(
home: new Scaffold(
appBar: new AppBar(
- title: const Text('Plugin example app'),
+ title: const Text('Flutter InAppBrowser Plugin example app'),
),
body: new Center(
child: new RaisedButton(onPressed: () {